Header slot
#header-{name}
:
You can customize only certain column header by using slot This is a new feature since version
1.2.25
<template>
<EasyDataTable
:headers="headers"
:items="items"
>
<template #header-name="header">
<div class="customize-header">
<img src="../images/name.png" class="header-icon">
{{ header.text }}
</div>
</template>
<template #header-address="header">
<div class="customize-header">
<img src="../images/address.png" class="header-icon">
{{ header.text }}
</div>
</template>
</EasyDataTable>
</template>
<script lang="ts" setup>
import { ref } from "vue";
import type { Header, Item } from "vue3-easy-data-table";
import { mockClientItems } from "../mock";
const items = ref<Item[]>(mockClientItems(100));
const headers: Header[] = [
{ text: "Name", value: "name" },
{ text: "Address", value: "address" },
{ text: "Height", value: "height", sortable: true },
{ text: "Weight", value: "weight", sortable: true },
{ text: "Age", value: "age", sortable: true },
{ text: "Favourite sport", value: "favouriteSport" },
{ text: "Favourite fruits", value: "favouriteFruits" },
];
</script>
<style>
.customize-header {
display: flex;
justify-items: center;
align-items: center;
}
.header-icon {
display: inline-block;
width: 20px;
height: 20px;
}
</style>
⚠️ Attention: the
{name}
of#header-{name}
should be a value of header item:
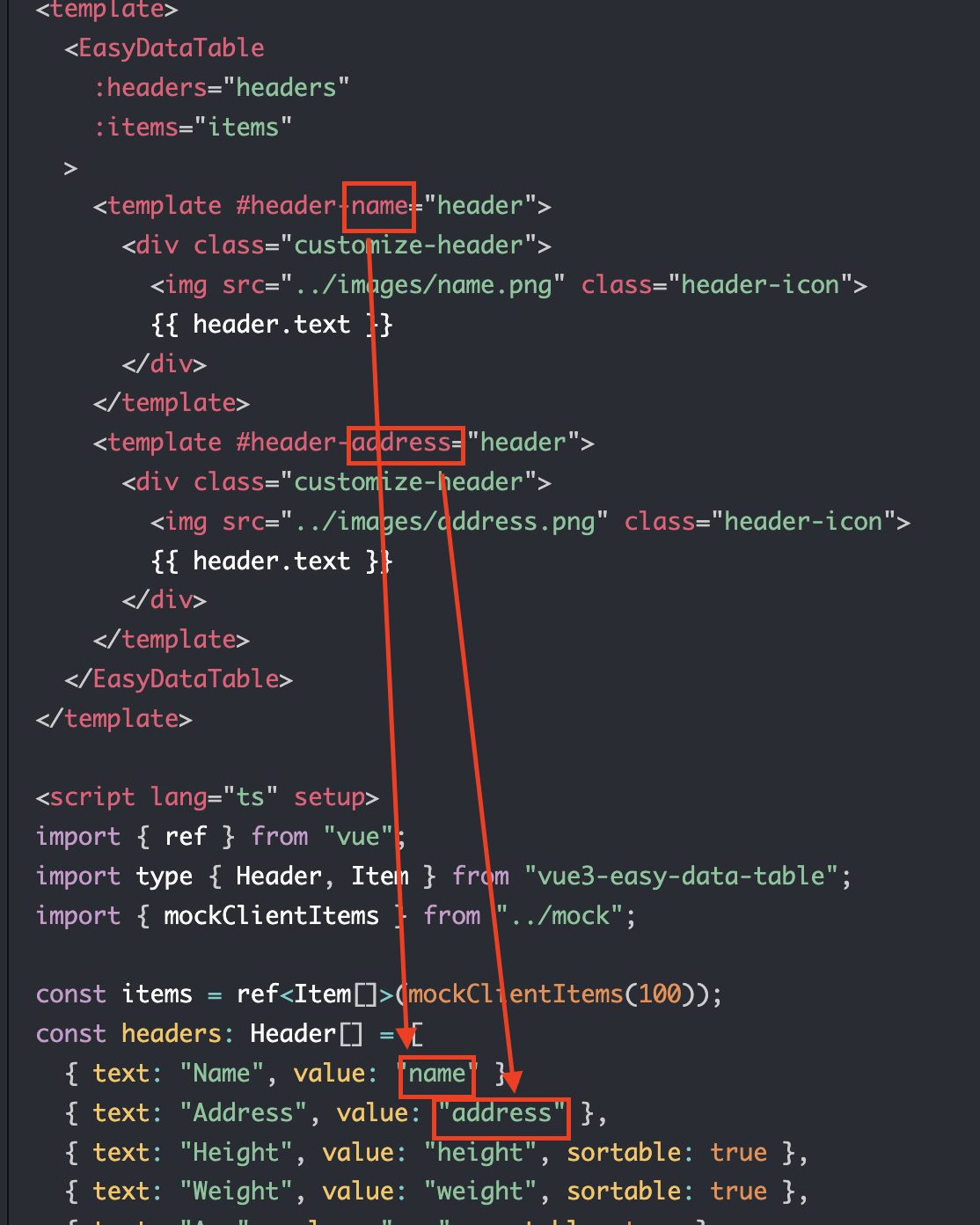
Example
# | Height | Weight | Age | Favourite sport | Favourite fruits | |||
---|---|---|---|---|---|---|---|---|
1 | name-1 | address-1 | 1 | 1 | 1 | football | apple | |
2 | name-2 | address-2 | 2 | 2 | 2 | running | orange | |
3 | name-3 | address-3 | 3 | 3 | 3 | swimming | peach | |
4 | name-4 | address-4 | 4 | 4 | 4 | basketball | banana | |
5 | name-5 | address-5 | 5 | 5 | 5 | football | apple | |
6 | name-6 | address-6 | 6 | 6 | 6 | running | orange | |
7 | name-7 | address-7 | 7 | 7 | 7 | swimming | peach | |
8 | name-8 | address-8 | 8 | 8 | 8 | basketball | banana | |
9 | name-9 | address-9 | 9 | 9 | 9 | football | apple | |
10 | name-10 | address-10 | 10 | 10 | 10 | running | orange | |
11 | name-11 | address-11 | 11 | 11 | 11 | swimming | peach | |
12 | name-12 | address-12 | 12 | 12 | 12 | basketball | banana | |
13 | name-13 | address-13 | 13 | 13 | 13 | football | apple | |
14 | name-14 | address-14 | 14 | 14 | 14 | running | orange | |
15 | name-15 | address-15 | 15 | 15 | 15 | swimming | peach | |
16 | name-16 | address-16 | 16 | 16 | 16 | basketball | banana | |
17 | name-17 | address-17 | 17 | 17 | 17 | football | apple | |
18 | name-18 | address-18 | 18 | 18 | 18 | running | orange | |
19 | name-19 | address-19 | 19 | 19 | 19 | swimming | peach | |
20 | name-20 | address-20 | 20 | 20 | 20 | basketball | banana | |
21 | name-21 | address-21 | 21 | 21 | 21 | football | apple | |
22 | name-22 | address-22 | 22 | 22 | 22 | running | orange | |
23 | name-23 | address-23 | 23 | 23 | 23 | swimming | peach | |
24 | name-24 | address-24 | 24 | 24 | 24 | basketball | banana | |
25 | name-25 | address-25 | 25 | 25 | 25 | football | apple |
header
slot to modify header text generically.
You can also use <template>
<EasyDataTable
:headers="headers"
:items="items"
>
<template #header="header">
{{ header.text.toUpperCase() }}
</template>
</EasyDataTable>
</template>
<script lang="ts" setup>
import { ref } from "vue";
import type { Header, Item } from "vue3-easy-data-table";
import { mockClientItems } from "../mock";
const items = ref<Item[]>(mockClientItems(100));
const headers: Header[] = [
{ text: "Name", value: "name" },
{ text: "Address", value: "address" },
{ text: "Height", value: "height", sortable: true },
{ text: "Weight", value: "weight", sortable: true },
{ text: "Age", value: "age", sortable: true },
{ text: "Favourite sport", value: "favouriteSport" },
{ text: "Favourite fruits", value: "favouriteFruits" },
];
</script>
Example
# | NAME | ADDRESS | HEIGHT | WEIGHT | AGE | FAVOURITE SPORT | FAVOURITE FRUITS | |
---|---|---|---|---|---|---|---|---|
1 | name-1 | address-1 | 1 | 1 | 1 | football | apple | |
2 | name-2 | address-2 | 2 | 2 | 2 | running | orange | |
3 | name-3 | address-3 | 3 | 3 | 3 | swimming | peach | |
4 | name-4 | address-4 | 4 | 4 | 4 | basketball | banana | |
5 | name-5 | address-5 | 5 | 5 | 5 | football | apple | |
6 | name-6 | address-6 | 6 | 6 | 6 | running | orange | |
7 | name-7 | address-7 | 7 | 7 | 7 | swimming | peach | |
8 | name-8 | address-8 | 8 | 8 | 8 | basketball | banana | |
9 | name-9 | address-9 | 9 | 9 | 9 | football | apple | |
10 | name-10 | address-10 | 10 | 10 | 10 | running | orange | |
11 | name-11 | address-11 | 11 | 11 | 11 | swimming | peach | |
12 | name-12 | address-12 | 12 | 12 | 12 | basketball | banana | |
13 | name-13 | address-13 | 13 | 13 | 13 | football | apple | |
14 | name-14 | address-14 | 14 | 14 | 14 | running | orange | |
15 | name-15 | address-15 | 15 | 15 | 15 | swimming | peach | |
16 | name-16 | address-16 | 16 | 16 | 16 | basketball | banana | |
17 | name-17 | address-17 | 17 | 17 | 17 | football | apple | |
18 | name-18 | address-18 | 18 | 18 | 18 | running | orange | |
19 | name-19 | address-19 | 19 | 19 | 19 | swimming | peach | |
20 | name-20 | address-20 | 20 | 20 | 20 | basketball | banana | |
21 | name-21 | address-21 | 21 | 21 | 21 | football | apple | |
22 | name-22 | address-22 | 22 | 22 | 22 | running | orange | |
23 | name-23 | address-23 | 23 | 23 | 23 | swimming | peach | |
24 | name-24 | address-24 | 24 | 24 | 24 | basketball | banana | |
25 | name-25 | address-25 | 25 | 25 | 25 | football | apple |
⚠️
header slot
feature is based on theslots
feature of vue.js. So before using theheader slot
feature in vue3-easy-data-table, Please make sure you have known how to use theslots
feature of vue.js.
customize-headers
slot
customize-headers
slot to customize headers like header grouping.
You can use ⚠️ Attention: If you are using
customize-headers
slot, you can not usesorting
feature,filtering
feature andfixed column
feature.
<template>
<EasyDataTable
:headers="headers"
:items="items"
/>
<template #customize-headers>
<thead class="customize-headers">
<tr>
<th colspan="2" rowspan="2"></th>
<th colspan="7">info</th>
</tr>
<tr>
<th>name</th>
<th>address</th>
<th>height</th>
<th>weight</th>
<th>age</th>
<th>favouriteSport</th>
<th>favouriteFruits</th>
</tr>
</thead>
</template>
</EasyDataTable>
</template>
Example
info | ||||||||
---|---|---|---|---|---|---|---|---|
name | address | height | weight | age | favouriteSport | favouriteFruits | ||
1 | name-1 | address-1 | 1 | 1 | 1 | football | apple | |
2 | name-2 | address-2 | 2 | 2 | 2 | running | orange | |
3 | name-3 | address-3 | 3 | 3 | 3 | swimming | peach | |
4 | name-4 | address-4 | 4 | 4 | 4 | basketball | banana | |
5 | name-5 | address-5 | 5 | 5 | 5 | football | apple | |
6 | name-6 | address-6 | 6 | 6 | 6 | running | orange | |
7 | name-7 | address-7 | 7 | 7 | 7 | swimming | peach | |
8 | name-8 | address-8 | 8 | 8 | 8 | basketball | banana | |
9 | name-9 | address-9 | 9 | 9 | 9 | football | apple | |
10 | name-10 | address-10 | 10 | 10 | 10 | running | orange | |
11 | name-11 | address-11 | 11 | 11 | 11 | swimming | peach | |
12 | name-12 | address-12 | 12 | 12 | 12 | basketball | banana | |
13 | name-13 | address-13 | 13 | 13 | 13 | football | apple | |
14 | name-14 | address-14 | 14 | 14 | 14 | running | orange | |
15 | name-15 | address-15 | 15 | 15 | 15 | swimming | peach | |
16 | name-16 | address-16 | 16 | 16 | 16 | basketball | banana | |
17 | name-17 | address-17 | 17 | 17 | 17 | football | apple | |
18 | name-18 | address-18 | 18 | 18 | 18 | running | orange | |
19 | name-19 | address-19 | 19 | 19 | 19 | swimming | peach | |
20 | name-20 | address-20 | 20 | 20 | 20 | basketball | banana | |
21 | name-21 | address-21 | 21 | 21 | 21 | football | apple | |
22 | name-22 | address-22 | 22 | 22 | 22 | running | orange | |
23 | name-23 | address-23 | 23 | 23 | 23 | swimming | peach | |
24 | name-24 | address-24 | 24 | 24 | 24 | basketball | banana | |
25 | name-25 | address-25 | 25 | 25 | 25 | football | apple |